access static members in java
Static members (variables and methods) in Java belong to the class rather than to any specific instance of the class. You can access static members using the class name followed by the dot (.) operator. Here’s how you can access static members in Java:
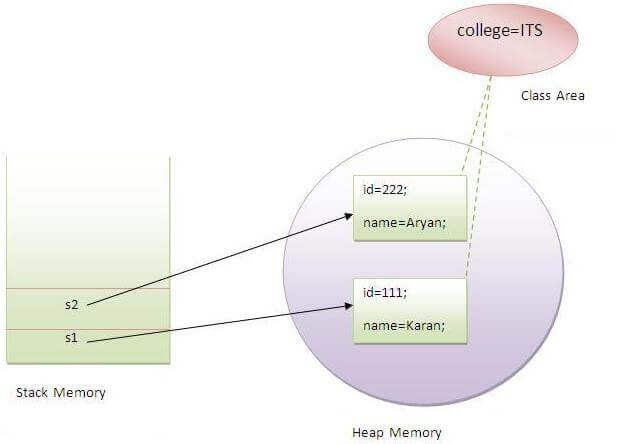
Table of Contents
1. Accessing Static Variables:
- You can access static variables directly using the class name followed by the dot (.) operator and the variable name.
- For example: `ClassName.staticVariable`.
2. Accessing Static Methods:
- You can invoke static methods using the class name followed by the dot (.) operator and the method name, followed by any required arguments.
- For example: `ClassName.staticMethod()`.
Here’s an example demonstrating how to access static members in Java:
Example
```java
public class MyClass {
// Static variable
public static int staticVar = 10;
// Static method
public static void staticMethod() {
System.out.println("Static method called");
}
public static void main(String[] args) {
// Accessing static variable
System.out.println("Static variable: " + MyClass.staticVar);
// Accessing static method
MyClass.staticMethod();
}
}
```
In this example:
- We access the static variable `staticVar` using `MyClass.staticVar`.
- We invoke the static method `staticMethod()` using `MyClass.staticMethod()`.