Custom Tags in JSP created
Note: below are Creating Custom Tags in JSP, give coding example for below tags
- Creating the Tag Handler Class
- Creating the TLD File
- Creating the JSP File
Custom tags in JSP are created using three main steps:
- Creating the Tag Handler Class
- Creating the Tag Library Descriptor (TLD) File
- Creating the JSP File
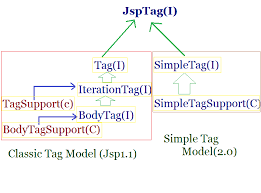
Table of Contents
Let’s go through each step with coding examples.
1. Creating the Tag Handler Class
The tag handler class defines the behavior of the custom tag. It extends TagSupport or BodyTagSupport and overrides the appropriate methods.
Example
Example: HelloTag.java
java
package com.example;
import javax.servlet.jsp.JspException;
import javax.servlet.jsp.tagext.TagSupport;
import java.io.IOException;
public class HelloTag extends TagSupport {
@Override
public int doStartTag() throws JspException {
try {
pageContext.getOut().print("Hello, Custom Tag!");
} catch (IOException e) {
throw new JspException("Error: " + e.getMessage());
}
return SKIP_BODY; // Skip the body content of the tag
}
}
2. Creating the Tag Library Descriptor (TLD) File
The TLD file describes the custom tags and maps them to their handler classes. Example: mytags.tld
Example
xml
<?xml version="1.0" encoding="UTF-8" ?>
<taglib xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-jsptaglibrary_2_1.xsd"
version="2.1">
<tlib-version>1.0</tlib-version>
<jsp-version>2.1</jsp-version>
<short-name>mytags</short-name>
<uri>http://example.com/tags</uri>
<tag>
<name>helloTag</name>
<tag-class>com.example.HelloTag</tag-class>
<body-content>empty</body-content>
</tag>
</taglib>
3. Creating the JSP File
The JSP file uses the custom tag by importing the tag library and then using the custom tag in the JSP page.
Example
Example: index.jsp
jsp
<%@ taglib uri="http://example.com/tags" prefix="ex" %>
<!DOCTYPE html>
<html>
<head>
<title>Custom Tag Example</title>
</head>
<body>
<h1>Using Custom Tags in JSP</h1>
<ex:helloTag />
</body>
</html>
Explanation
1. Creating the Tag Handler Class :
- The HelloTag class extends TagSupport.
- The doStartTag method is overridden to define the custom behavior of the tag. In this case, it prints “Hello, Custom Tag!” to the JSP page’s output.
- The method returns SKIP_BODY to indicate that the tag does not have a body.
2. Creating the TLD File :
- Â The mytags.tld file defines the tag library.
- Â It specifies the tag name (helloTag), the handler class (com.example.HelloTag), and the body content type (empty).
3. Creating the JSP File :
- Â The index.jsp file imports the tag library using the <%@ taglib %> directive.
- Â It uses the custom tag <ex:helloTag /> to include the output defined in the HelloTag class.