deploy microservices in Docker
Deploying microservices in Docker involves containerizing each microservice, managing their dependencies, and orchestrating their interaction. This approach simplifies deployment, scaling, and management of microservices. Here’s an overview of the steps involved:
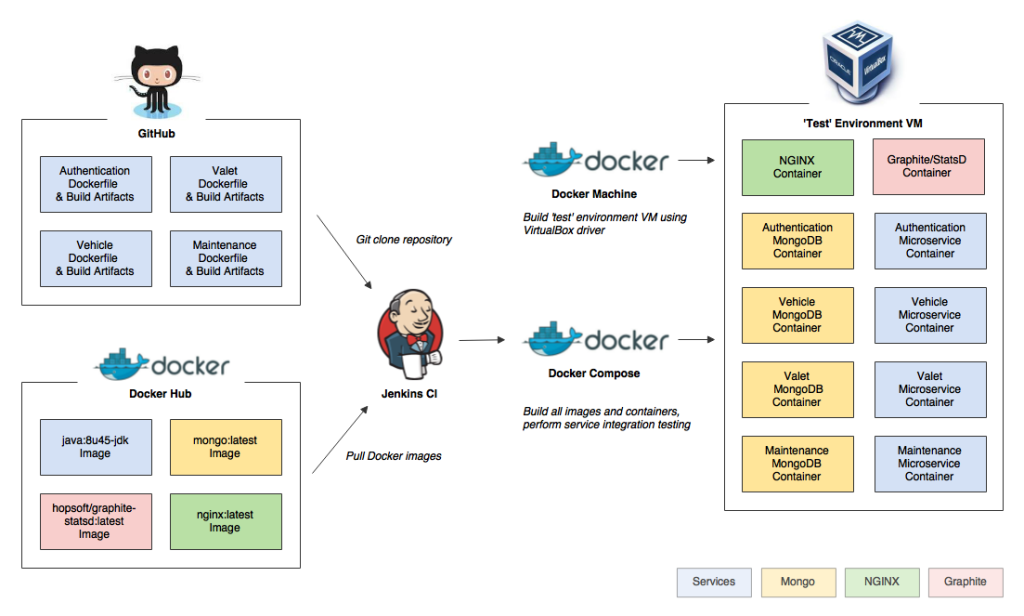
Table of Contents
- 1. Containerize Each Microservice: Create Docker images for each microservice, defining their dependencies and runtime environment.
- 2. Docker Compose for Orchestration: Use Docker Compose to define and manage multi-container Docker applications. This allows you to specify how the microservices interact, including networking, environment variables, and volume mounts.
- 3. Deploy to a Container Orchestrator: Use Docker Swarm or Kubernetes for managing and scaling your Docker containers in production. These tools provide features like load balancing, scaling, and rolling updates.
Java Example with Docker Compose
Let’s go through a practical example of deploying two Java microservices using Docker and Docker Compose.
- 1. Create Microservices
- Service A: A simple REST API
Example
```java
package com.example.servicea;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
public class ServiceAApplication {
public static void main(String[] args) {
SpringApplication.run(ServiceAApplication.class, args);
}
}
@RestController
class HelloController {
@GetMapping("/hello")
public String sayHello() {
return "Hello from Service A";
}
}
```
- Create a `Dockerfile` for Service A
Example
```dockerfile
FROM openjdk:11-jdk
COPY target/service-a-0.0.1-SNAPSHOT.jar app.jar
EXPOSE 8081
ENTRYPOINT ["java", "-jar", "/app.jar"]
```
Service B: Another simple REST API
```java
package com.example.serviceb;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
public class ServiceBApplication {
public static void main(String[] args) {
SpringApplication.run(ServiceBApplication.class, args);
}
}
@RestController
class HelloController {
@GetMapping("/hello")
public String sayHello() {
return "Hello from Service B";
}
}
```
Create a `Dockerfile` for Service B
```dockerfile
FROM openjdk:11-jdk
COPY target/service-b-0.0.1-SNAPSHOT.jar app.jar
EXPOSE 8082
ENTRYPOINT ["java", "-jar", "/app.jar"]
```
- 2. Build Docker Images
- Navigate to each service’s directory and build the Docker images:
Example
```bash
docker build -t service-a .
docker build -t service-b .
```
- 3. Docker Compose Configuration
- Create a `docker-compose.yml` file to define both services:
Example
```yaml
version: '3.8'
services:
service-a:
image: service-a
ports:
- "8081:8081"
service-b:
image: service-b
ports:
- "8082:8082"
```
- 4. Run the Docker Compose Setup
- Start the services with Docker Compose:
Example
```bash
docker-compose up -d
```
- 5. Verify the Deployment
- Check the status of the containers:
Example
```bash
docker-compose ps
```
Access the services through your browser or `curl`
```bash
curl http://localhost:8081/hello
curl http://localhost:8082/hello
```
You should see responses indicating that Service A and Service B are running.
Summary
Using Docker and Docker Compose, you can easily deploy and manage microservices. Docker provides isolation and consistency, while Docker Compose simplifies orchestration. This setup is scalable, making it suitable for both development and production environments.