Explain @Configuration annotation
The `@Configuration` annotation in Spring is used to indicate that a class declares one or more `@Bean` methods. These `@Bean` methods define the beans that will be managed by the Spring IoC (Inversion of Control) container. The `@Configuration` annotation is a part of the Spring Framework and is used to create Java-based configuration for Spring applications, as opposed to XML-based configuration.
Using `@Configuration`, you can create a configuration class that provides a centralized place to define all the beans and configuration settings for your Spring application.
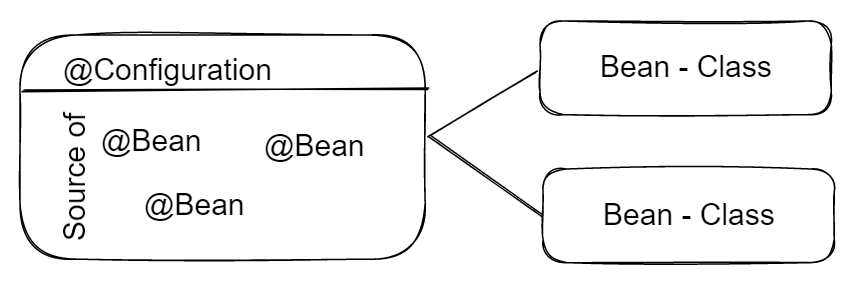
Table of Contents
Example of Configuration annotation
Step 1: Create a Configuration Class
Create a class and annotate it with `@Configuration`. Inside this class, define methods annotated with `@Bean` to declare the beans managed by the Spring container.
```java
package com.example.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class AppConfig {
@Bean
public MyService myService() {
return new MyServiceImpl();
}
@Bean
public MyRepository myRepository() {
return new MyRepositoryImpl();
}
}
```
Explanation:
@Configuration
: Indicates that the class can be used by the Spring IoC container as a source of bean definitions.@Bean
: Indicates that a method produces a bean to be managed by the Spring container
Step 2: Create Service and Repository Interfaces and Implementations
Define the interfaces and their implementations.
```java
package com.example.service;
public interface MyService {
String serve();
}
package com.example.service;
public class MyServiceImpl implements MyService {
@Override
public String serve() {
return "Service is running!";
}
}
```
```java
package com.example.repository;
public interface MyRepository {
String getData();
}
package com.example.repository;
public class MyRepositoryImpl implements MyRepository {
@Override
public String getData() {
return "Repository data";
}
}
```
Explanation
- Defined a service interface
MyService
and its implementationMyServiceImpl
. - Defined a repository interface
MyRepository
and its implementationMyRepositoryImpl
.
Step 3: Using the Configuration in Application
In your main application class or any other class, you can now inject these beans.
```java
package com.example.demo;
import com.example.service.MyService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DemoApplication implements CommandLineRunner {
@Autowired
private MyService myService;
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
@Override
public void run(String... args) throws Exception {
System.out.println(myService.serve());
}
}
```
Explanation:
@Autowired
: Used to inject theMyService
bean defined inAppConfig
.- The
run
method prints the message returned by theserve
method ofMyService
.
Conclusion of Configuration annotation
- @Configuration: Indicates that a class can be used as a source of bean definitions. It allows for a centralized way to define all the beans and configuration settings for your Spring application.
- Example: Demonstrated how to use
@Configuration
to define beans and how to use these beans in a Spring Boot application.