Explain @RequestMapping annotation
The `@RequestMapping` annotation in Spring is used to map web requests to specific handler methods in your controller. It allows you to specify the URL patterns and request methods (e.g., GET, POST) that a method should handle. This annotation is a fundamental part of Spring MVC and provides a way to define and manage web request mappings in a flexible manner.
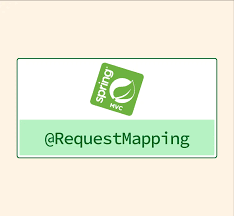
Table of Contents
Example
Step 1: Create a Spring Boot Application
Ensure you have the necessary dependencies in your `pom.xml` (for Maven) or `build.gradle` (for Gradle).
Maven Dependency:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
Gradle Dependency:
```groovy
implementation 'org.springframework.boot:spring-boot-starter-web'
```
Step 2: Create the Main Application Class
Create the main class for the Spring Boot application.
```java
package com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
```
Explanation:
@SpringBootApplication
: Indicates a configuration class that declares one or more@Bean
methods and also triggers auto-configuration and component scanning.
Step 3: Create a Controller
Create a controller class that uses the @RequestMapping
annotation to handle different types of HTTP requests.
```java
package com.example.demo.controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/api")
public class HelloController {
@RequestMapping(value = "/hello", method = RequestMethod.GET)
public String sayHello() {
return "Hello, World!";
}
@RequestMapping(value = "/greet", method = RequestMethod.POST)
public String greet() {
return "Greetings!";
}
@RequestMapping(value = "/welcome/{name}", method = RequestMethod.GET)
public String welcome(String name) {
return "Welcome, " + name + "!";
}
}
```
Explanation:
@RestController
: Indicates that this class is a controller and the return values of methods are directly written to the HTTP response body.@RequestMapping("/api")
: Specifies that all methods in this class handle requests starting with/api
.@RequestMapping(value = "/hello", method = RequestMethod.GET)
: Maps GET requests to/api/hello
to thesayHello
method.@RequestMapping(value = "/greet", method = RequestMethod.POST)
: Maps POST requests to/api/greet
to thegreet
method.@RequestMapping(value = "/welcome/{name}", method = RequestMethod.GET)
: Maps GET requests to/api/welcome/{name}
to thewelcome
method.{name}
is a path variable.
Step 4: Running the Application
Run the application from the main class (`DemoApplication`). You can test the endpoints using a tool like Postman or by navigating to the URLs in your browser:
- GET request to `http://localhost:8080/api/hello` should return “Hello, World!”.
- POST request to `http://localhost:8080/api/greet` should return “Greetings!”.
- GET request to `http://localhost:8080/api/welcome/John` should return “Welcome, John!”.
Conclusion of RequestMapping annotation
- `@RequestMapping`: Used to map web requests to specific handler methods in a controller.
- Example: Demonstrated how to use `@RequestMapping` to handle different types of HTTP requests and URL patterns.