How to use JSTL with Spring MVC?
 JSTL is a library of custom tags used in JavaServer Pages (JSP) to simplify common tasks such as iteration, conditionals, and formatting. It provides a standard set of tags that help developers avoid scripting in JSP.
Usage:
JSTL tags are used to perform common tasks directly within JSP pages, improving readability and maintainability of the code.
Steps to Use JSTL with Spring MVC:
1. Add JSTL Dependency: Include JSTL in your project’s dependencies.
2. Configure JSTL in JSP: Import JSTL tags into your JSP pages.
3. Use JSTL Tags: Utilize JSTL tags in your JSP to perform various tasks.
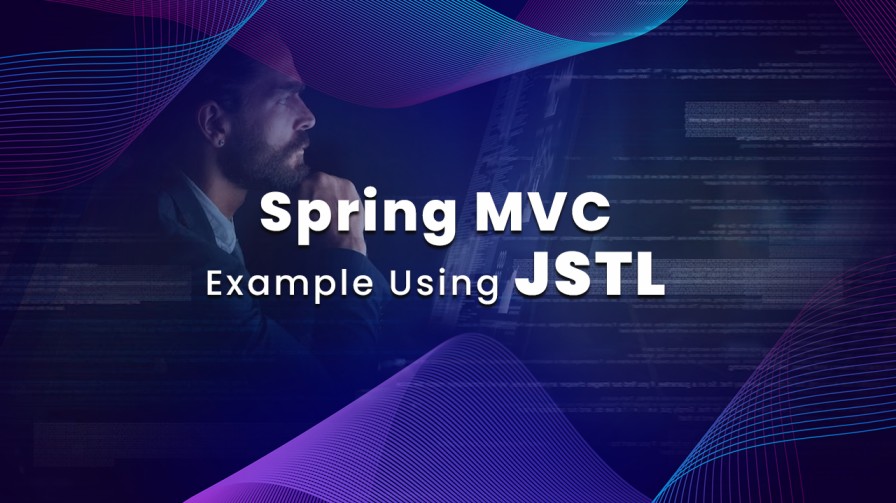
Table of Contents
Example of JSTL with Spring MVC
1. Add JSTL Dependency
To use JSTL in your Spring MVC project, you need to add the JSTL library to your project. If you are using Maven, add the following dependency to your `pom.xml`:
pom.xml
```xml
<dependencies>
<!-- Other dependencies -->
<!-- JSTL dependency -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
</dependencies>
```
2. Configure JSTL in JSP
Include JSTL tag libraries in your JSP pages. The most common JSTL tags are found in the c
(core) and fmt
(format) libraries.
example.jsp
```jsp
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/fmt" prefix="fmt" %>
<html>
<head>
<title>JSTL Example</title>
</head>
<body>
<h2>JSTL Core Tags Example</h2>
<!-- JSTL Core Tag: c:out -->
<p>Hello, <c:out value="${userName}" />!</p>
<!-- JSTL Core Tag: c:if -->
<c:if test="${userLoggedIn}">
<p>Welcome back, <c:out value="${userName}" />!</p>
</c:if>
<!-- JSTL Core Tag: c:forEach -->
<h3>Item List:</h3>
<ul>
<c:forEach var="item" items="${items}">
<li><c:out value="${item}" /></li>
</c:forEach>
</ul>
<!-- JSTL Formatting Tag: fmt:formatDate -->
<fmt:formatDate value="${currentDate}" pattern="yyyy-MM-dd" />
</body>
</html>
```
In this example:
<c:out>
: Outputs the value of theuserName
variable.<c:if>
: Displays content if theuserLoggedIn
variable evaluates to true.<c:forEach>
: Iterates over theitems
list and displays each item.<fmt:formatDate>
: Formats thecurrentDate
variable according to the specified pattern.
3. Pass Data to JSP from Controller
Ensure that your Spring MVC controller sets the required model attributes for use in the JSP.
UserController.java
```java
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import java.util.Arrays;
import java.util.Date;
import java.util.List;
@Controller
public class UserController {
@GetMapping("/example")
public String example(Model model) {
model.addAttribute("userName", "John Doe");
model.addAttribute("userLoggedIn", true);
List<String> items = Arrays.asList("Item 1", "Item 2", "Item 3");
model.addAttribute("items", items);
model.addAttribute("currentDate", new Date());
return "example";
}
}
```
In this example:
model.addAttribute
: Adds attributes (userName
,userLoggedIn
,items
, andcurrentDate
) to the model, which can be accessed in the JSP page.
Summary
- JSTL: Provides standard tags to simplify JSP development.
Steps
- Add Dependency: Include JSTL library in your project.
- Configure JSP: Import JSTL tag libraries in JSP pages.
- Use Tags: Utilize JSTL tags for common tasks like iteration and conditionals.
- Example:
- Demonstrates usage of JSTL tags (
c:out
,c:if
,c:forEach
,fmt:formatDate
) in JSP, and passing data from a Spring MVC controller.
- Demonstrates usage of JSTL tags (