log4j logging in hibernate application
Log4j is a popular logging framework for Java applications that provides a reliable and flexible way to log application messages. Integrating Log4j with Hibernate helps in logging Hibernate-related activities such as SQL statements, transaction events, and other operational messages. This can be very useful for debugging and monitoring purposes.
Steps to Integrate Log4j with Hibernate:
- Include Log4j Dependency : Add the Log4j dependency to your project.
- Configure Log4j : Create a log4j.properties file to configure logging levels and appenders.
- Configure Hibernate Logging : Update Hibernate configuration to use Log4j for logging.
- Use Log4j in Your Application : Utilize Log4j in your Java classes for logging messages.
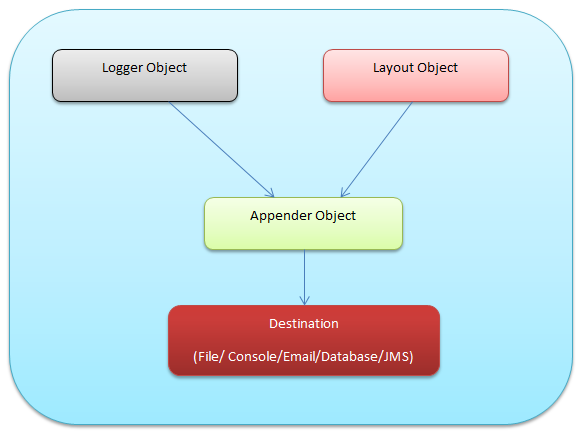
Table of Contents
Example of log4j logging in hibernate application
Let’s go through the steps to integrate Log4j logging into a Hibernate application.
Step 1: Include Log4j Dependency
Add the Log4j dependency to your project. If you are using Maven, add the following dependency to your pom.xml file:
xml
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
Step 2: Configure Log4j
Create a log4j.properties file in the src/main/resources directory:
properties
Root logger option
log4j.rootLogger=DEBUG, stdout, file
Redirect log messages to console
log4j.appender.stdout=org.apache.log4j.ConsoleAppender
log4j.appender.stdout.Target=System.out
log4j.appender.stdout.layout=org.apache.log4j.PatternLayout
log4j.appender.stdout.layout.ConversionPattern=%d{yyyy-MM-dd HH:mm:ss} %-5p %c{1}:%L - %m%n
Redirect log messages to a log file
log4j.appender.file=org.apache.log4j.RollingFileAppender
log4j.appender.file.File=logs/hibernate.log
log4j.appender.file.MaxFileSize=5MB
log4j.appender.file.MaxBackupIndex=10
log4j.appender.file.layout=org.apache.log4j.PatternLayout
log4j.appender.file.layout.ConversionPattern=%d{yyyy-MM-dd HH:mm:ss} %-5p %c{1}:%L - %m%n
Hibernate logging configuration
log4j.logger.org.hibernate=DEBUG
log4j.logger.org.hibernate.type=TRACE
Step 3: Configure Hibernate Logging
Update the Hibernate configuration file (hibernate.cfg.xml) to enable SQL logging and format SQL statements for better readability:
xml
<!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<!-- Database connection settings -->
<property name="hibernate.connection.driver_class">com.mysql.cj.jdbc.Driver</property>
<property name="hibernate.connection.url">jdbc:mysql://localhost:3306/mydb</property>
<property name="hibernate.connection.username">root</property>
<property name="hibernate.connection.password">password</property>
<!-- JDBC connection pool settings ... using built-in test pool -->
<property name="hibernate.c3p0.min_size">5</property>
<property name="hibernate.c3p0.max_size">20</property>
<property name="hibernate.c3p0.timeout">300</property>
<property name="hibernate.c3p0.max_statements">50</property>
<property name="hibernate.c3p0.idle_test_period">3000</property>
<!-- Specify dialect -->
<property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property>
<!-- Update the database schema on startup -->
<property name="hibernate.hbm2ddl.auto">update</property>
<!-- Enable Hibernate SQL logging -->
<property name="hibernate.show_sql">true</property>
<property name="hibernate.format_sql">true</property>
<property name="hibernate.use_sql_comments">true</property>
<!-- Mappings -->
<mapping class="com.example.Product"/>
</session-factory>
</hibernate-configuration>
Step 4: Use Log4j in Your Application
In your Java classes, you can use Log4j for logging messages. Here's an example:
java
import org.apache.log4j.Logger;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class Main {
private static final Logger logger = Logger.getLogger(Main.class);
public static void main(String[] args) {
// Set up the Hibernate session factory
SessionFactory sessionFactory = new Configuration().configure().buildSessionFactory();
Session session = null;
try {
session = sessionFactory.openSession();
session.beginTransaction();
// Create and save a Product
Product product = new Product();
product.setId(1);
product.setName("Laptop");
product.setPrice(1500.00);
session.save(product);
// Commit the transaction
session.getTransaction().commit();
logger.info("Product saved successfully: " + product);
} catch (Exception e) {
if (session != null) {
session.getTransaction().rollback();
}
logger.error("Transaction failed", e);
} finally {
if (session != null) {
session.close();
}
sessionFactory.close();
logger.info("Session factory closed");
}
}
}
Explanation of the Example
- Include Log4j Dependency : The Log4j dependency is added to the project.
- Configure Log4j : The log4j.properties file is created to define logging levels, appenders, and formatting.
- Configure Hibernate Logging : The Hibernate configuration file is updated to enable SQL logging and format SQL statements.
- Use Log4j in Your Application : Log4j is utilized in the main application class to log messages during different stages of the transaction.
This example demonstrates how to integrate Log4j logging into a Hibernate application, providing a structured way to log Hibernate activities and other application messages.