Serialize and deSerialize object
Serialization in Java involves converting an object into a byte stream that can be persisted (e.g., saved to a file) or transmitted over a network. Deserialization is the process of reconstructing an object from its serialized byte stream. This process is useful for tasks like data persistence, caching, and inter-process communication.
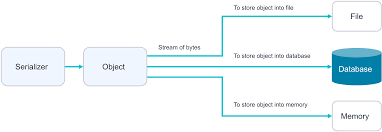
Table of Contents
Steps to Serialize and Deserialize an Object
1. Make the Class Serializable: Ensure that the class whose objects you want to serialize implements the Serializable interface. This is a marker interface, meaning it does not contain any methods but indicates that the objects of this class can be serialized.
2. Serialization: Use ObjectOutputStream to serialize the object and write it to a file or any output stream.
3. Deserialization: Use ObjectInputStream to read the serialized object from a file or input stream and reconstruct it back into an object Serialize and deSerialize.
Let’s see a Java program that demonstrates serialization and deserialization of an object.
Serializable Class Example
java
import java.io.Serializable;
public class Employee implements Serializable {
private static final long serialVersionUID = 1L;
private String name;
private int age;
private double salary;
public Employee(String name, int age, double salary) {
this.name = name;
this.age = age;
this.salary = salary;
}
@Override
public String toString() {
return "Employee{name='" + name + "', age=" + age + ", salary=" + salary + "}";
}
}
java
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
public class SerializeEmployee {
public static void main(String[] args) {
Employee employee = new Employee("John Doe", 30, 50000.0);
try (FileOutputStream fileOut = new FileOutputStream("employee.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut)) {
out.writeObject(employee);
System.out.println("Serialized data is saved in employee.ser");
} catch (IOException i) {
i.printStackTrace();
}
}
}
3. Deserialization Example:
java
import java.io.FileInputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
public class DeserializeEmployee {
public static void main(String[] args) {
Employee employee = null;
try (FileInputStream fileIn = new FileInputStream("employee.ser");
ObjectInputStream in = new ObjectInputStream(fileIn)) {
employee = (Employee) in.readObject();
} catch (IOException | ClassNotFoundException i) {
i.printStackTrace();
}
System.out.println("Deserialized Employee:");
System.out.println(employee);
}
}
Explanation of the Example
- Serializable Class (Employee)
- The Employee class implements the Serializable interface, indicating that objects of this class can be serialized.
- It has fields like name, age, and salary.
- Serialization (SerializeEmployee):
- The SerializeEmployee class creates an Employee object and serializes it to a file named employee.ser.
- ObjectOutputStream.writeObject(Object obj) is used to write the object to the file.
- Deserialization (DeserializeEmployee)
- The DeserializeEmployee class reads the Employee object from the employee.ser file using ObjectInputStream.readObject().
- The deserialized object is then printed to the console.