struts-default package and benefits
In Struts2, the struts-default package is a built-in package provided by the framework. It is defined in the Struts2 core library and includes a collection of commonly used interceptors and result types. This package is intended to simplify the configuration process by providing a default set of functionalities that can be extended or overridden as needed.
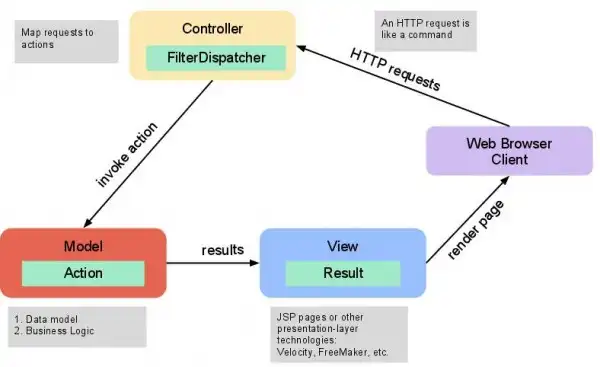
Table of Contents
Benefits of Using Struts-Default Package:
- 1. Â Convenience : Provides a pre-configured set of interceptors and result types, reducing the amount of configuration required.
- 2. Â Reusability : Common functionalities such as validation, file upload handling, and exception handling are included, making them easily reusable across actions.
- 3. Â Standardization : Promotes a standard way of configuring actions and interceptors, leading to more consistent code.
- 4. Â Extensibility : Developers can extend the struts-default package to add custom interceptors or result types while still leveraging the default functionalities.
Java Example
Let’s create a simple Struts2 application using the struts-default package.
Step 1: Configure Struts2 with the Default Package
xml
<!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<!-- Define a package that extends the struts-default package -->
<package name="default" namespace="/" extends="struts-default">
<!-- Define an action -->
<action name="hello" class="com.example.action.HelloAction">
<result name="success">/hello.jsp</result>
</action>
</package>
</struts>
Step 2: Create Struts2 Action
java
package com.example.action;
import com.opensymphony.xwork2.ActionSupport;
public class HelloAction extends ActionSupport {
private String message;
@Override
public String execute() {
message = "Hello, World!";
return SUCCESS;
}
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
}
Step 3: Create JSP Page
jsp
<%@ taglib uri="/struts-tags" prefix="s" %>
<html>
<head>
<title>Hello</title>
</head>
<body>
<h1><s:property value="message" /></h1>
</body>
</html>
Explanation of the Example
- 1. Â Configuration : The struts.xml file defines a package named default that extends struts-default. This means the package inherits all the interceptors and result types defined in struts-default.
- 2. Â Struts2 Action : HelloAction.java is a simple action that sets a greeting message.
- 3. Â JSP Page : hello.jsp displays the message set by HelloAction.
Benefits in the Example:
- Convenience : By extending struts-default, we automatically inherit the default set of interceptors, which includes functionalities like parameter handling, validation, and exception handling.
- Reusability : If we have other actions, they can also extend from the same default package, reusing the same set of interceptors and configurations.
- Standardization : Using the struts-default package ensures that our configuration follows a standard structure, making it easier for other developers to understand and maintain.