What is Spring Initializr with example?
Spring Initializr is a web-based tool provided by the Spring team to bootstrap a Spring project quickly. It allows developers to generate a ready-to-run Spring Boot application with the necessary dependencies and configurations. It simplifies the setup process and helps developers get started with Spring projects without manually creating the project structure and configuration files.
Features of Spring Initializr:
- Dependency Management: Choose from a wide range of dependencies such as Web, JPA, Security, etc.
- Project Metadata: Configure project metadata like Group, Artifact, Name, Description, Package Name, etc.
- Build Tool Selection: Choose between Maven or Gradle as the build tool.
- Packaging Options: Select the packaging type (JAR or WAR).
- Java Version: Choose the desired Java version for the project.
Steps to Use Spring Initializr:
- Access Spring Initializr: Open your web browser and navigate to [start.spring.io](https://start.spring.io).
- Configure Project Metadata: Fill in the fields like Group, Artifact, Name, Description, Package Name, etc.
- Select Dependencies: Choose the necessary dependencies for your project (e.g., Spring Web, Spring Data JPA, H2 Database).
- Generate the Project: Click the “Generate” button to download a ZIP file containing the project.
- Import into IDE: Extract the ZIP file and import the project into your favorite IDE (e.g., IntelliJ IDEA, Eclipse).
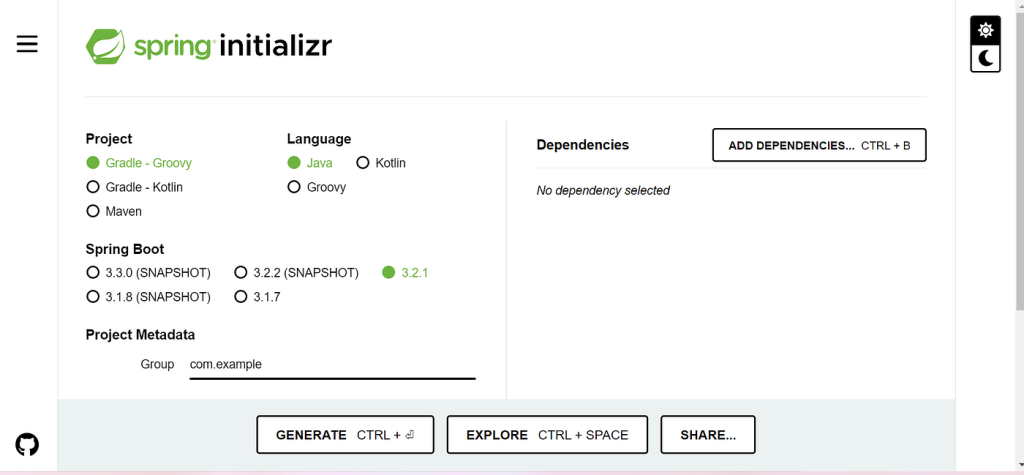
Table of Contents
Example
Let’s go through an example of creating a Spring Boot project using Spring and adding a simple REST controller.
1. Access Spring Initializ:
  Go to [start.spring.io](https://start.spring.io).
2. Configure Project Metadata:
- Â Group: `com.example`
- Â Â Artifact: `demo`
- Â Â Name: `Demo`
- Â Â Description: `Demo project for Spring Boot`
- Â Â Package Name: `com.example.demo`
- Â Â Packaging: JAR
- Â Â Java: 11
3. Select Dependencies:
- Â Â Spring Web
- Â Â Spring Data JPA
- Â Â H2 Database
4. Generate the Project:
  Click “Generate” and download the project ZIP file.
5. Import into IDE:
  Extract the ZIP file and import the project into your IDE.
Java Code Example
MainApplication.java
```java
package com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MainApplication {
public static void main(String[] args) {
SpringApplication.run(MainApplication.class, args);
}
}
```
application.properties
```properties
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=password
spring.jpa.database-platform=org.hibernate.dialect.H2Dialect
```
User.java
```java
package com.example.demo;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String name;
// Getters and Setters
}
```
UserRepository.java
```java
package com.example.demo;
import org.springframework.data.repository.CrudRepository;
public interface UserRepository extends CrudRepository<User, Long> {
}
```
UserController.java
```java
package com.example.demo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping("/users")
public Iterable<User> getAllUsers() {
return userRepository.findAll();
}
}
```
Summary
- Spring Initializr: A web-based tool to bootstrap Spring Boot projects quickly.
- Features: Allows configuration of project metadata, selection of dependencies, and generation of a ready-to-run Spring Boot project.
- Example: Demonstrates creating a Spring Boot project with a simple REST controller using Spring-Initializr.