finalize method in java
The finalize
method in Java is a method provided by the Object
class. It’s called by the garbage collector before an object is reclaimed (i.e., before it is garbage-collected) to perform cleanup operations. The primary purpose of the finalize
method is to allow an object to release resources or perform other cleanup actions before it is no longer reachable.
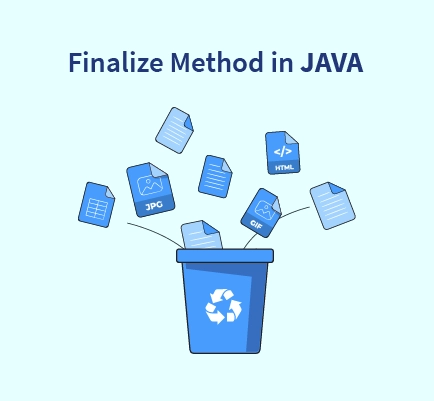
Here’s a brief overview of the finalize
method and its usage:
1. Method Signature:
- The
finalize
method has the following signature in theObject
class:
protected void finalize() throws Throwable;
2. Usage:
- When an object is no longer reachable (i.e., there are no references to it), the garbage collector identifies it as a candidate for garbage collection.
- Before the object is actually reclaimed, the
finalize
method is called if it’s overridden in the class of the object.
3. Cleanup Operations:
- The
finalize
method can be used to perform cleanup operations such as closing files, releasing network resources, or any other activities that need to be completed before the object is garbage-collected.
4. Caution and Best Practices:
- While the
finalize
method provides an opportunity for cleanup, it’s important to note that relying on it for critical resource management is not recommended. - The timing of when
finalize
is called is not guaranteed, and there is no certainty about when the garbage collector will run. - Modern Java applications often use other mechanisms, like try-with-resources for resource management, which provide more predictable behavior.
5. Deprecated in Java 9:
- In Java 9, the
finalize
method has been deprecated with a warning that it is often misused and is inherently problematic. The recommendation is to use other mechanisms for resource management.
Here’s a simple example to illustrate the use of finalize
:
class ResourceHolder {
// Some resource or state to be cleaned up
private String resource;
public ResourceHolder(String resource) {
this.resource = resource;
}
@Override
protected void finalize() throws Throwable {
try {
// Cleanup operations, e.g., closing a file
System.out.println("Finalizing: " + resource);
} finally {
// Call the finalize method of the superclass
super.finalize();
}
}
}
public class FinalizeExample {
public static void main(String[] args) {
// Creating an object that requires cleanup
ResourceHolder resourceObj = new ResourceHolder("File.txt");
// Making the object eligible for garbage collection
resourceObj = null;
// Explicitly triggering garbage collection (for illustration purposes)
System.gc();
}
}
In this example, the finalize
method of the ResourceHolder
class is called before the object is garbage-collected, allowing for cleanup operations to be performed. Keep in mind that relying on finalize
is generally discouraged, and alternative resource management techniques are preferred in modern Java development.